C# Delegate and Event
Description
Delegate and event are good ways to “subscribe” some actions to other particular actions carried out by the user or the code. It sounds like function 1 is executed firstly, then function 2, 3, and etc are executed following. Those functions may not in the same class.
For example, they are in class A, class B, class C. If we use the traditional ways to achieve this relationship, we need to call function 2 and 3 in the class A when the function 1 is executed. In this way we will increase the degree of coupling between these classes, that is class A cannot work normally without class B and C.
Therefore, we proposed the ideas of delegate and event. Class B and C could “subscribe” the action publisher in class A with their function 2 and 3. When the action 1 is executed, the class A will call the action publisher, then the class B and C will receive the information that action 1 is executed, so they will execute the action 2 and 3.
Comparison between delegate and event
- Commons
- They are like the pointer of methods.
- A method can be used += and -= to be assigned or unassigned to a delegate, and subscribe or unsubscribe the event
- They both accept anonymous methods (delegate declared, or lambda expression)
- Event is a specific kind of delegate which will be safer
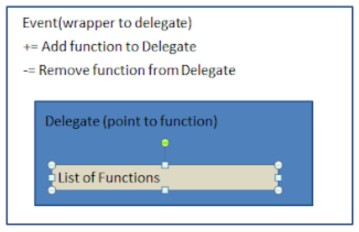
- Differences
delegate | event |
---|---|
Functions can use += to subscribe it, and -= to unsubscribe it, and = to fully occupy the delegate | Only support += and -= |
Is a type, still need to instantiate a member | Is a variable instance |
Can be defined outside the class | Can only be defined inside the class |
Can be executed outside the class | Only can be executed in the class where it defines |
delegate is the member of the class (static member) | event belongs to the instance of a class |
Can be invoked outside the class | Cannot be invoked outside the class |
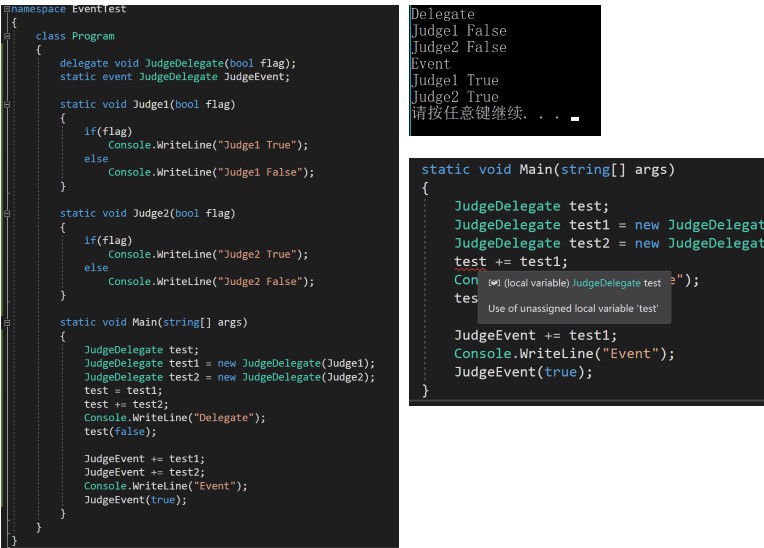
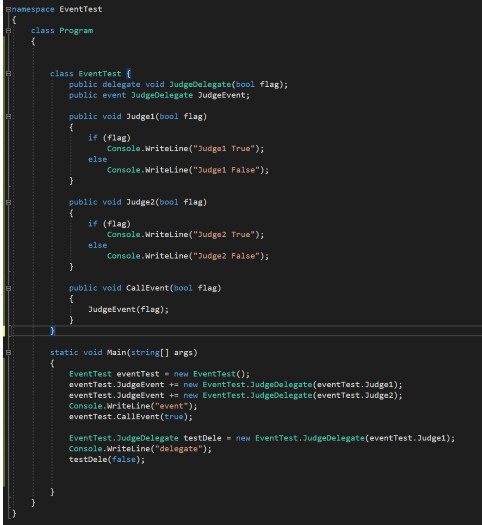
Generic delegate
1 | event EventHandler<bool> OnEvent1; |
Delegate declaring anonymous methods
Delegate can be used to declare an anonymous method.
1 | event MyHandler<EventArgs> OnTrigger; |
See more about anonymous method from here
Execution
The delegate and event can both be used two methods to executed.
- Use
myDelegateInstance(paras)
ormyEvent(paras)
- Use
myDelegateInstance.Invoke(paras)
ormyEvent.Invoke(paras)
They are the same in compiler but the second method could add ?
to handle the null conditions.
myDelegateInstance?.Invoke(paras)
or myEvent?.Invoke(paras)