C++ Overview
General introduction of C++ related knowledge.
Overview
What is C++
C++ is a programming language. ISO is responsible for making and maintaining its language standards.
Standards of C++
C++ 98/03, C++ 11, C++ 14, C++ 17, C++ 20
Each C++ standards consists of the
- C++ features and functionalities, like lambda expression
- C++ API, a collection of classes, functions and macros, also called standard library
- mainly describes the library interface and the efficiency requirement
- includes some function libraries and STL (Standard Template Library)
For more details about C++ syntax and standard library usages, refer this post.
Implementation of C++
C++ is a compiled language, meaning it needs compiler to translate into machine code, for execution or get used as a library.
Each platform implements their own compiler and standard libraries for each C++ language feature standards.
Platform (OS) | Compiler Implementation | Standard Library Implementation |
---|---|---|
Windows (x86, x64, ARM) | MSVC (Microsoft Visual C++) | MSVCRT, MinGW |
Linux (x86, x64, ARM) | GCC (GNU Compiler Collection) | glibc |
macOS (x86, x64, ARM) | Clang | libc++ |
More insights about standard libraries
- Standard library is tightly coupled with Operation System because they need to convert the OS’s function into C++ interface. e.g MSVCRT needs to depend on Windows API (win32), and glibc needs to depend on Linux System Call.
- In Windows platform, the compiler and standard libraries are usually aggregated into Visual Studio (IDE). But developers could also use other libraries and standard libraries implementation like MinGw. The MinGW refactors the glibc libraries by replacing Linux System Call with Windows API.
Therefore, a C++ project needs to set the target platform and the language standard version, then uses respective compiler to compile and depends on respective standard libraries.
Compile Link Process
C++ uses the compile link model to convert source code to binary machine code for execution. The compile process requires lots of resources but the number of input files are usually small. The link process has lots of files input but the processing speed is fast.
- Preprocess: interpret the macro, convert source code to “interpret unit” (source code + related header files - preprocess commands)
- e.g. interpret
#pragma once
- e.g. interpret
- Compile: convert interpret unit to object files
- Cpp Compiler: convert to assembly code
- Assembler: covert to binary file
- Link: combine with other library files
Clang compile process
Different compiler has their own compiling process. Clang in macOS use a 3-steps process and uses the LLVM (Low-Level Virtual Machine) as the compile backend.
Clang separate the compile process into
- Frontend: analyze syntax of source code, generate IR (Intermediate Representation)
- Optimizer: optimize IR code
- Backend: convert IR into machine code according to specific OS architecture
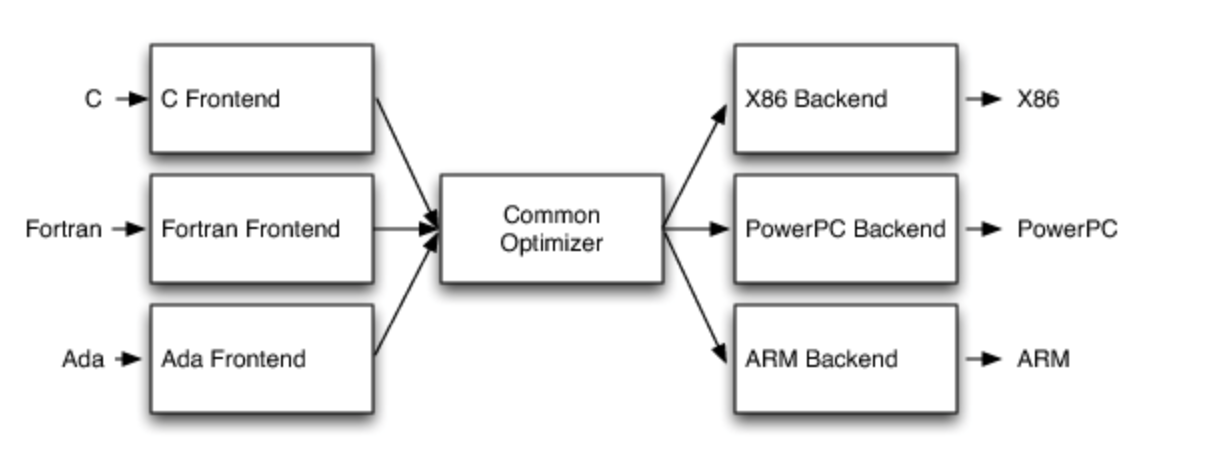
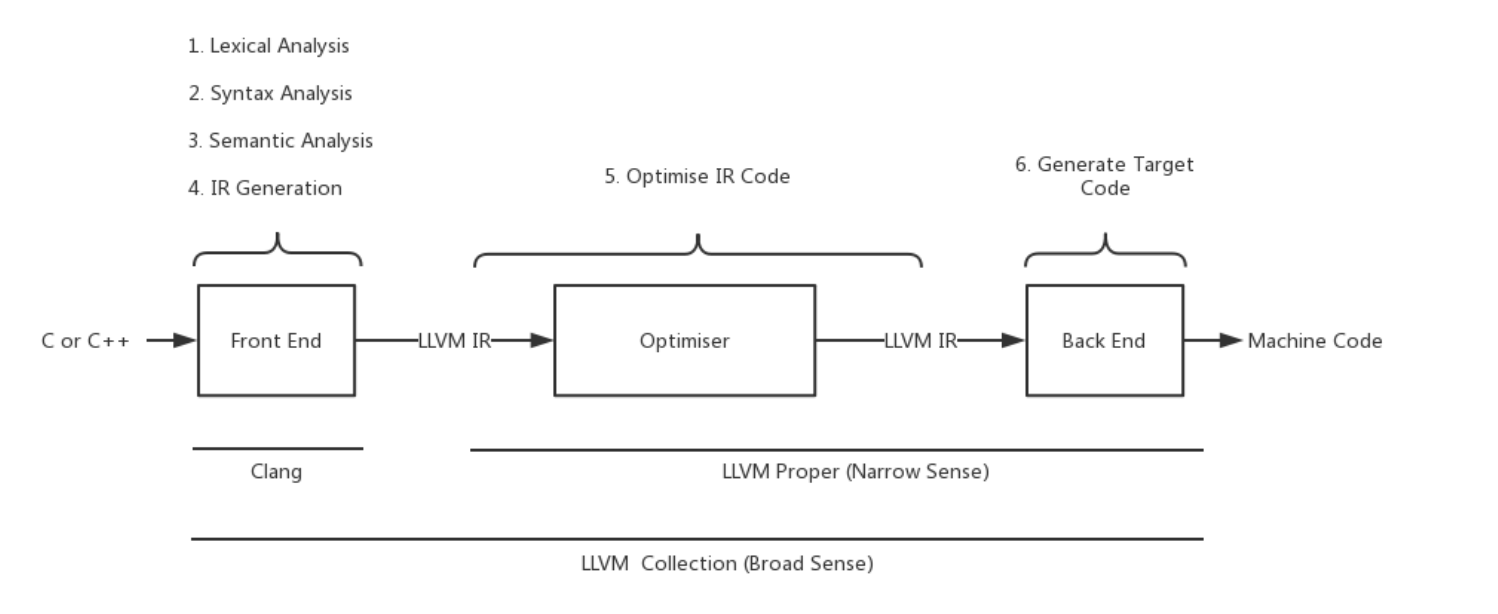
Strengths
- High performance
- Stay closely with underline hardware: different platforms may have different results
- Control the lifecycle of the object accurately
- Zero-overhead abstraction
- Don’t need to pay for unused features e.g. virtual function tables won’t be generated for classes don’t used virtual functions
- Don’t need to pay during runtime for some language features
- For large projects
- Process oriented, object oriented, generics oriented
- Function override, exception handling, reference type
Dialects
Dialects is the standard C++ with some extensions.
Name | Description |
---|---|
C++/CLI | - extension of the C++ - includes new keywords such as the ref new and ^ (hat) notation |
C++/CX | - adds non-standard extensions to the C++ language - inherited syntax from C++/CLI |
Library
Library is a collection of non-volatile resources used by computer programs. These may include configuration data, documentation, help data, message templates, pre-written code and subroutines, classes, values or type specifications. A library is also a collection of implementations of behavior, written in terms of a language, that has a well-defined interface by which the behavior is invoked.
WRL (Windows Runtime C++ Template Library, Legacy). WRL is exception-free, meaning its return-value discipline is HRESULT-based just like that of COM.
C++/WinRT is an entirely standard C++ language projection (the way that Win32 uses to present APIs) for Microsoft’s Windows Runtime (WinRT) platform, designed to provide access to modern Windows APIs, implemented as a header-file-based library. With C++/WinRT, developers can author and consume Windows Runtime APIs using any standards-compliant C++17 compiler. Github link
C++/WinRT is standard C++, so we cannot use it to determine the application type. Both Win32 applications and UWP applications are able to use C++/WinRT to call WinRT APIs.
Boost library is also a famous library for C++, which is usually regarded as a pre-standard library.
Build System
When the C++ project is too large so using compiler directly is not convenient, build system needs to be used.
Some common-used build systems include
Make
: GNU build tool. UsedMakefile
to execute the building process.ninja
: build system focusing on build efficiency, especially when doing incremental build. Usingbuild.ninja
Both Makefile
and build.ninja
files are hard to write directly, so build system generator like CMake
, which uses human-friendly CMakeList.txt
, needs to be used to generate them.
Therefore, the whole building process for a large C++ project are as follows.
- Write
CMakeList.txt
files withCMake
syntax to configure the projects - Use
CMake
to generateMakefile
orbuild.ninja
files- Could specify which one to use like
cmake -G ninja
- Could specify which one to use like
- Use
Make
orninja
to build the files using different compilers